Introduction
Choosing the right way to organize your app's code is essential in iOS development, where innovation and efficiency are key. This decision impacts how easy it is to maintain, scale, and test your applications. Among the various design patterns available, two main ones are often used: Model-View-Controller (MVC) and Model-View-ViewModel (MVVM). Many beginners find it confusing to decide which coding pattern to use. Figuring out when to use each one can be a bit unclear, adding an extra layer of complexity for those who are just starting out.
In this exploration, we'll dive into these architectures, looking at their strengths, possible drawbacks, and core ideas, all within the context of Swift, the language that powers iOS development. To start our journey, let's take a close look at the Model-View-Controller (MVC) architecture. This approach has been a fundamental part of iOS app development for quite some time.
Model-View-Controller (MVC) Architecture
Definition
The Model-View-Controller (MVC) architecture is a fundamental approach in iOS development that simplifies how we build applications. It breaks down the app into three key components, each with a specific role:
- Model: This is like the brain of the operation. The Model manages all the data and the essential logic of the application. It ensures that information is stored correctly and that the app's core processes run smoothly. For instance, if you're building a to-do list app, the Model would handle tasks, due dates, and any related functions.
- View: Imagine this as the part of the app that you actually see and interact with – the user interface. The View is responsible for displaying data from the Model in a way that makes sense to users. Going back to the to-do list example, the View would show a list of tasks, checkboxes, and maybe a due date next to each task.
- Controller: Think of the Controller as the coordinator or director. It takes input from the user (like tapping a button or entering text) and communicates with the Model to make things happen accordingly. For our to-do list, the Controller would handle tasks like adding a new item, marking a task as complete, or deleting a task. It then tells the View to update and show the changes.
In essence, MVC provides a clear structure for organising code, making it easier to manage and understand. It promotes a separation of concerns, ensuring that each component does its job without getting too entangled with the others. This separation enhances maintainability, scalability, and collaboration among developers working on different aspects of the app.
Here is a visual representation of how the MVC architecture works:

Advantages:
- Simplicity: MVC's straightforward structure makes it easy to understand and implement, especially for smaller projects or when a minimalistic approach is preferred.
- Clear Separation of Concerns: The distinct roles assigned to Model, View, and Controller ensure a clean separation of concerns, fostering modularity and maintainability.
Drawbacks:
- Massive View Controllers: As projects grow in complexity, there is a risk of Controllers accumulating numerous responsibilities, resulting in Massive View Controllers that are challenging to maintain and test.
- Tangled Dependencies: The close coupling between Model, View, and Controller may lead to tangled dependencies, making it harder to modify or extend specific components without affecting others.
- Limited Testability: The tightly coupled nature of MVC can hinder unit testing, as testing individual components in isolation becomes challenging due to the interconnected nature of Model, View, and Controller.
- Limited Support for Reactive Programming: MVC might struggle to accommodate reactive programming paradigms efficiently, making it less adaptable to modern approaches in iOS development.
In summary, while MVC's simplicity is advantageous for certain scenarios, its drawbacks become more pronounced in larger projects, prompting developers to explore alternative architectures like Model-View-ViewModel (MVVM) to address these limitations.
Let us now look at a simple implementation of the MVC architecture so you have a clear idea about when and how to use it.
Implementation
Model (User.swift)
View (UserProfileView.swift)
Controller (UserProfileViewController.swift)
Now that we have discussed Model-View-Controller Architecture in detail, let us now have a thorough understanding of the Model-View-ViewModel (MVVM) architecture, and see how it is different from the MVC architecture.
Model-View-ViewModel (MVVM) Architecture
Definition:
The Model-View-ViewModel (MVVM) architecture is like the refined version of iOS development, introducing a ViewModel as a mediator between the View and the Model. Let's delve into its components:
- Model: This is where all the crucial information lives. The Model handles the data and the rules that govern how the application functions. It acts as the brains behind the scenes, much like it does in the traditional MVC architecture.
- View: This is what users interact with – the buttons, text, and visual elements. The View's primary role is to showcase the data stored in the Model, as seen in MVC.
- ViewModel: Here comes the new player. The ViewModel is like an assistant, managing how data from the Model is presented in the View. It takes care of the visual aspects, ensuring data is well-prepared for display. Moreover, the ViewModel handles user interactions, such as button clicks or text entries, acting as a bridge between the Model and the View.
So, in MVVM, the Model continues to handle data, the View continues to display things, but now the ViewModel steps in to refine how the data appears and responds to user actions. It's akin to having a helper to streamline the presentation and functionality aspects, contributing to a more organised and comprehensible code structure. This extra layer introduced by MVVM enhances the clarity and maintainability of your codebase.
Advantages:
- Enhanced Testability: MVVM excels in testability by reducing dependencies between components. The ViewModel, serving as an interface between the Model and View, can be easily unit tested in isolation.
- Improved Maintainability: With the ViewModel taking on presentation logic, ViewControllers become more streamlined, mitigating the risk of Massive View Controllers. This separation of concerns enhances code maintainability and readability.
- Reactive Programming Support: MVVM seamlessly integrates with reactive programming paradigms, providing a more responsive and efficient way to handle data binding and asynchronous updates.
- Loose Coupling: MVVM fosters loose coupling between components, making it easier to modify or extend specific functionalities without affecting other parts of the application.
Drawbacks:
While MVVM offers significant advantages, it's essential to acknowledge potential drawbacks:
- Learning Curve: Adopting MVVM might pose a learning curve for developers not familiar with the architectural pattern, especially when transitioning from traditional MVC.
- Boilerplate Code: Some implementations of MVVM may introduce additional boilerplate code, potentially impacting codebase verbosity.
- Overhead: For smaller projects, the introduction of a ViewModel layer might be perceived as unnecessary overhead, potentially outweighing the benefits.
In conclusion, MVVM stands as a robust architectural pattern, addressing many of the drawbacks associated with MVC. Its emphasis on testability, maintainability, and loose coupling makes it a compelling choice for modern iOS development, especially in scenarios where scalability and responsiveness are paramount.
Similar to the MVC architecture, here’s a small implementation of the MVVM architecture for better understanding.
Implementation
Model
ViewModel
View
Key Concepts of MVVM
Although in many ways, the MVVM architecture can be considered as a refinement over the MVC architecture, there might be scenarios where the MVC architecture would be a better option for executing the app flow and organising code. However, there are a few key concepts involved in the MVVM architecture that we must know about.
Data Binding
One of the hallmark features of MVVM is its robust implementation of data binding, facilitating a dynamic and responsive user interface. In the MVVM pattern, the View and ViewModel are bound together, creating a live connection that allows changes in one to be reflected in the other. Two-way data binding, in particular, streamlines the process of updating the UI based on changes in the underlying data and vice versa. This bidirectional communication ensures that the user interface remains synchronised with the ViewModel, offering a seamless and intuitive user experience.
Dependency Injection
MVVM promotes the use of dependency injection as a fundamental concept to foster loose coupling between different components. Dependency injection involves providing a component with its dependencies from an external source rather than having the component create those dependencies itself. In the context of MVVM, this means that the ViewModel can be injected with its dependencies, such as services or repositories, making the codebase more modular and testable. This decoupling of components simplifies unit testing, as it becomes easier to substitute dependencies with mock objects, ensuring that tests focus on individual components without unnecessary entanglements.
Tools and Libraries
Swift developers embracing MVVM often leverage specialized libraries to streamline and enhance the implementation of this architectural pattern. Two prominent libraries in the Swift ecosystem are RxSwift and Combine.
- RxSwift: RxSwift is a reactive programming library for Swift that enables developers to work with asynchronous data streams. It complements MVVM by providing a reactive approach to data binding and event handling. Observables and Bindings in RxSwift seamlessly integrate with MVVM, simplifying the propagation of changes between the View and ViewModel.
- Combine: Introduced by Apple, Combine is a framework that brings reactive programming to the Swift language. It provides a declarative Swift API for processing values over time. Combine is designed to work seamlessly with MVVM, offering publishers and subscribers that align well with the data binding principles of this architectural pattern.
The incorporation of these tools and libraries into the MVVM architecture empowers Swift developers to build more responsive, scalable, and maintainable applications, especially when dealing with complex asynchronous events and user interface updates. The choice between RxSwift and Combine often depends on project requirements and team preferences, as both libraries excel in enhancing the reactive capabilities of MVVM.
The Choice: Choosing Between MVVM and MVC
The decision-making process between adopting the Model-View-ViewModel (MVVM) or Model-View-Controller (MVC) architectural pattern is a critical aspect of iOS development. Several factors come into play, influencing the suitability of each pattern based on the unique characteristics of the project.
Project Size and Complexity
- MVC for Simplicity: In scenarios where the project is relatively small or follows a simple structure, MVC might be the preferable choice. Its simplicity and ease of implementation make it well-suited for projects where a lightweight architectural pattern is sufficient to meet the application's requirements.
- MVVM for Scalability: As the size and complexity of the project increase, MVVM shines as a more scalable solution. MVVM's introduction of the ViewModel layer, separating concerns and handling presentation logic, makes it particularly adept at managing intricate user interfaces and complex business logic without succumbing to the pitfalls of Massive View Controllers.
Team Familiarity and Expertise
- MVC for Familiarity: If the development team is more accustomed to traditional patterns or has extensive experience with MVC, sticking to familiarity might be pragmatic. This ensures a smoother onboarding process for new team members and reduces the learning curve associated with transitioning to a new architectural paradigm.
- MVVM for Modern Practices: For teams looking to embrace modern iOS development practices and take advantage of reactive programming, MVVM provides a forward-looking approach. The use of tools like RxSwift or Combine aligns well with contemporary development trends, offering enhanced flexibility and responsiveness.
Testability and Maintainability
- MVC Challenges: MVC, especially in larger projects, may face challenges related to the testability of components and the maintainability of code. The risk of Massive View Controllers and tightly coupled dependencies can impede the effectiveness of unit testing and overall code maintainability.
- MVVM Advantages: MVVM addresses these challenges by promoting loose coupling, making it easier to write unit tests for individual components. The ViewModel layer serves as a mediator, reducing dependencies and enhancing the overall maintainability of the codebase.
Conclusion
In conclusion choosing between MVVM and MVC isn't a one-size-fits-all decision. It's more about what makes sense for the specific project. MVC is still great for smaller projects or if your team is used to traditional patterns. On the other hand, MVVM really shines in bigger, more intricate projects. It brings scalability, easier testing, and a more modern way of doing things in iOS development. So, the key is to think about what your project needs, what your team is comfortable with, and making a smart decision that aligns with the best practices in iOS development.
Send us a quick query
Recommended Posts
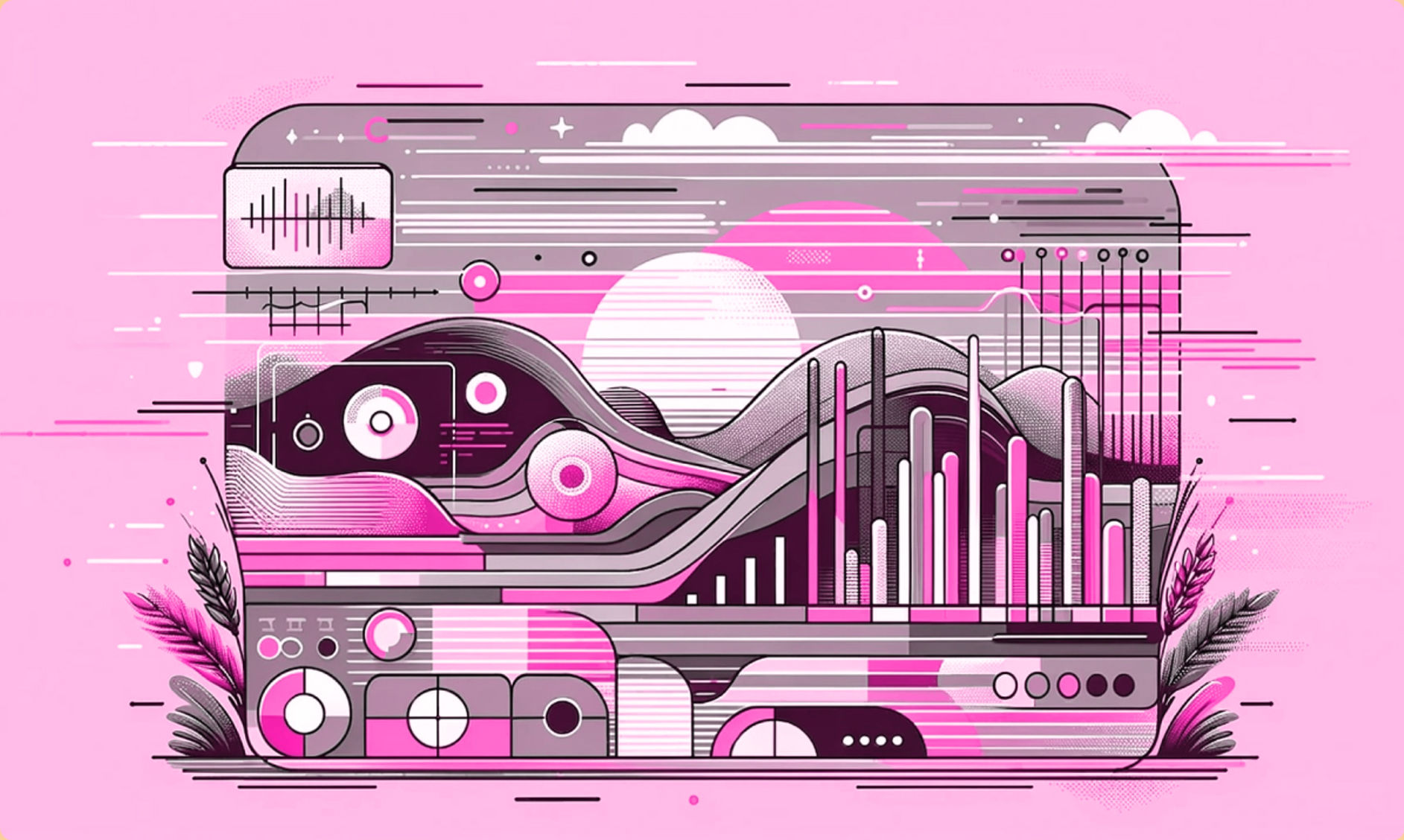
UI Performance Testing Guide In 2023 for Front-end Developers
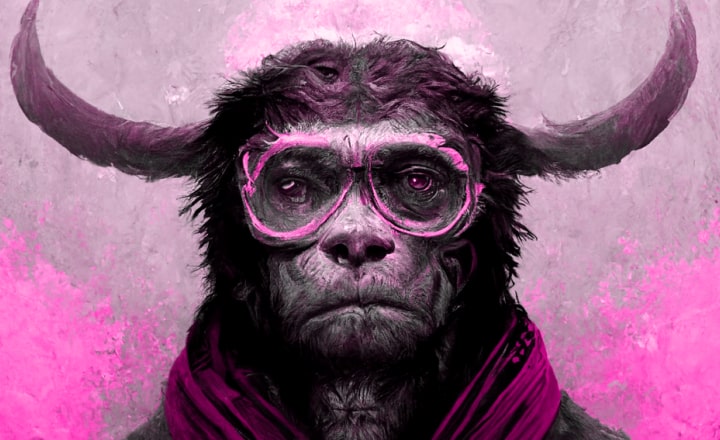
Could 'Prompting' be the future of creativity?
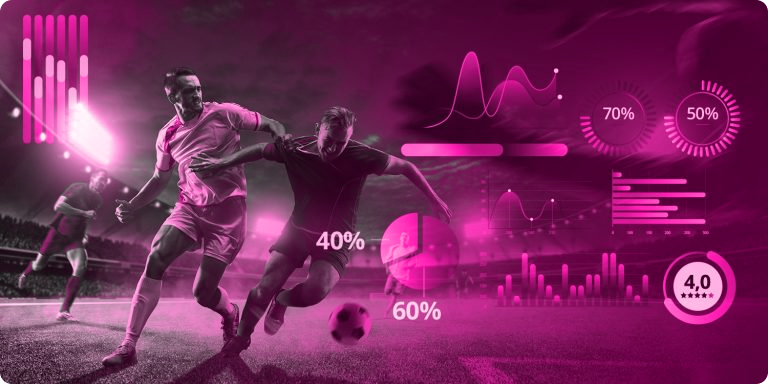