After the acquisition of FitBit, Google set out to create a toolkit that would act as a central health data repository on Android, mimicking Apple’s HealthKit. The FitBit API will be officially deprecated at the end of 2024, and from Android 14 onward, Google’s new platform, called Health Connect is the official way to go when dealing with health data.
An introduction
Health Connect is a health data platform for Android app developers. It provides a single, consolidated interface for access to users' health and fitness data and consistent functional behavior across all devices. With Health Connect, users have a secure health and fitness data storage on-device, with full control and transparency over access.
From Google’s Codelab on Health Connect
Essentially, Google has created a unified ecosystem for managing health data such as steps, heart rate, sleep, and others.
If you’re a fitness app developer, what this means for you is that now you have to use the Health Connect platform to store and sync your app data instead of using local storage. There are standardized data types, and since all apps will be doing I/O on the health connect platform, you can get access to data from other apps as well, and use it to create better plans. Permissions are also simplified, and it’s much easier to get started.
If you’re a user, it means better privacy and centralized access to your health data sourced from different devices. You can own a Samsung Gear watch and a FitBit, and see data from both devices in one place using Health Connect. Essentially, all of your fitness apps start talking with each other, giving you ease of access.
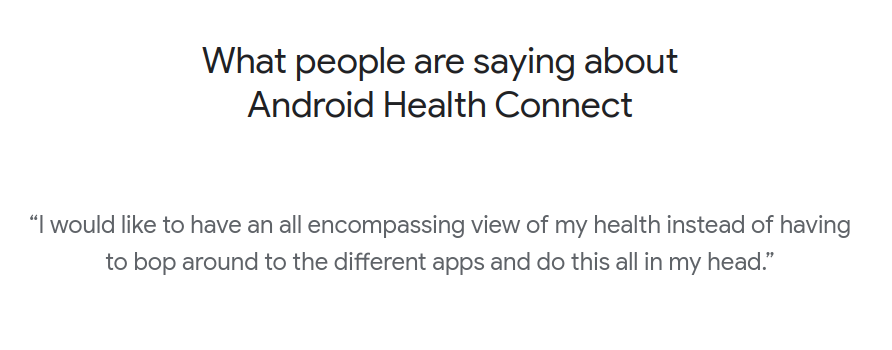
Availability and Adoption
The Health Connect app works on Android 9 onward and is currently in open beta on the Play Store. Updated Pixel devices should already have the app installed.
It’s important to note that Health Connect will become part of Android 14 as an Android system app, so switching to the platform is essential if you’re a health app developer.
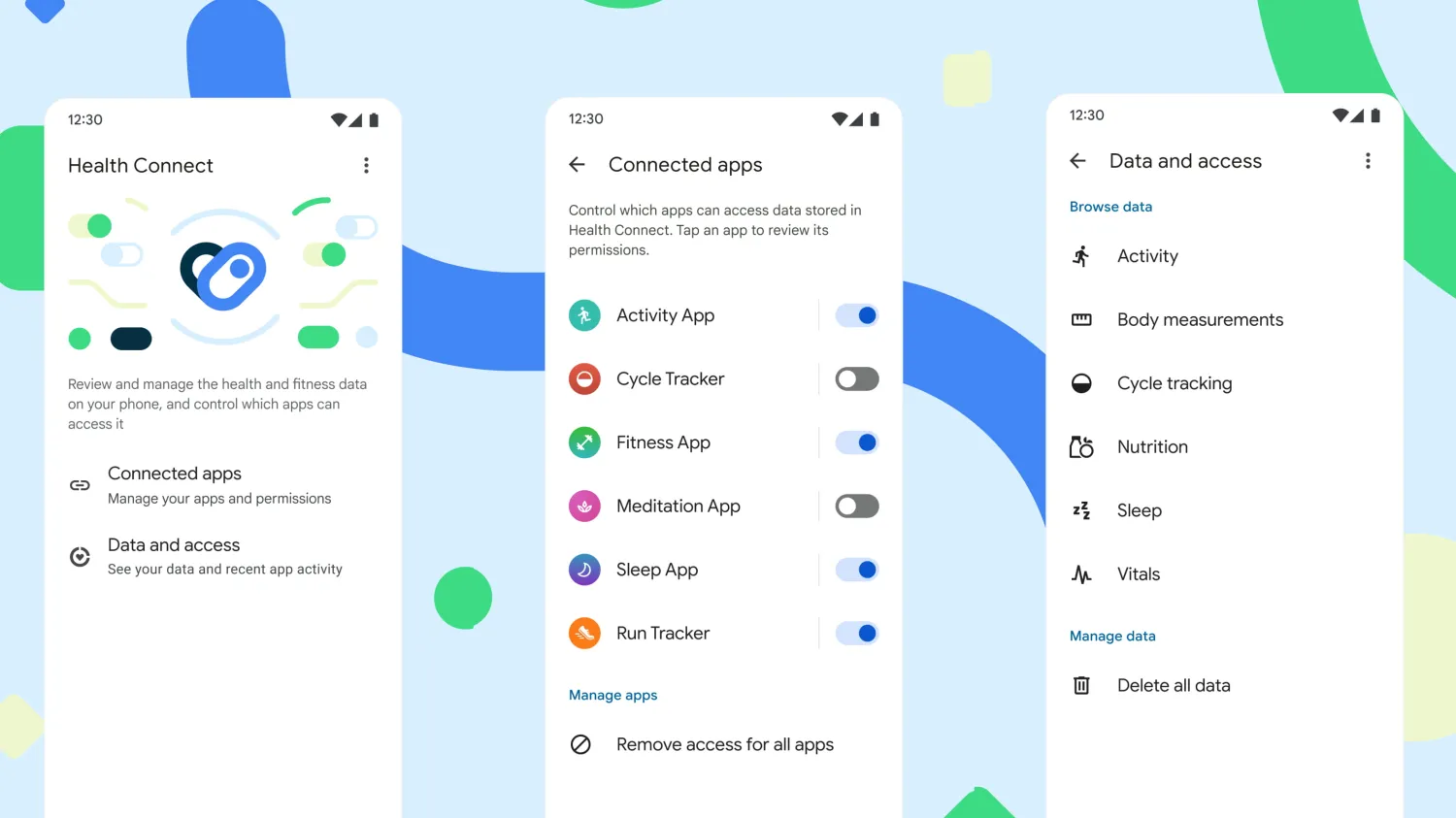
There are currently over 100 apps that have already integrated with the Health Connect platform. This includes major players like Samsung Health, Fitbit, Withings, MyFitnessPal and Peloton.
Getting Started
To use the Health Connect platform, there are 2 requirements:
- Presence of the Health Connect app on the device. Available on Play Store
- Integration of your fitness app with the Health Connect SDK
Once you’ve finished these steps, you can start using the Health Connect platform in your apps via these steps:
Step 1: Check whether the Health Connect app is available on the device.
Define package query in your AndroidManifest.xml
<queries>
<package android:name="com.google.android.apps.healthdata" />
</queries>
[add copy]
Check whether the app is installed or not
val availabilityStatus = HealthConnectClient.sdkStatus(context, "your.package.name")
if (availabilityStatus == HealthConnectClient.SDK_UNAVAILABLE) {
return // early return as there is no viable integration
}
if (availabilityStatus == HealthConnectClient.SDK_UNAVAILABLE_PROVIDER_UPDATE_REQUIRED) {
val uriString = "https://play.google.com/store/apps/details?id=com.google.android.apps.healthdata"
context.startActivity(Intent(Intent.ACTION_VIEW, uriString.toUri()))
return
}
val healthConnectClient = HealthConnectClient.getOrCreate(context)
[add copy]
Step 2: Handle permissions
Declare permissions, see list of types here
<uses-permission android:name="android.permission.health.READ_STEPS"/>
<uses-permission android:name="android.permission.health.WRITE_STEPS"/>
[add copy]
Provide a rationale activity that describes your privacy policy and how you’ll use your data. You may need to create an additional activity for this.
<application>
...
<!-- Activity to show rationale of Health Connect permissions -->
<activity
android:name=".RationaleActivity"
android:exported="true"
android:enabled="true">
<intent-filter>
<action android:name="androidx.health.ACTION_SHOW_PERMISSIONS_RATIONALE" />
</intent-filter>
</activity>
...
</application>
...
[add copy]
Ask for permissions on runtime
val PERMISSIONS =
setOf(
HealthPermission.getReadPermission(StepsRecord::class),
HealthPermission.getWritePermission(StepsRecord::class)
)
val requestPermissionActivityContract = PermissionController.createRequestPermissionResultContract()
val requestPermissions =
registerForActivityResult(requestPermissionActivityContract) { granted ->
if (granted.containsAll(PERMISSIONS)) {
// Permissions successfully granted
} else {
// Permission Denied
}
}
suspend fun checkPermissionsAndRun(healthConnectClient: HealthConnectClient) {
val granted = healthConnectClient.permissionController.getGrantedPermissions()
if (granted.containsAll(PERMISSIONS)) {
// Permissions already granted; proceed with inserting or reading data.
} else {
requestPermissions.launch(PERMISSIONS)
}
}
[add copy]
If the user denies these permissions for your app twice, the permission popup will no longer appear. Instead, you’re gonna have to guide them to enable permissions in the Health Connect app itself. So you might want to add some permission rationale here as well.
Data I/O
There are currently 39 distinct data types available with the Health Connect platform, with more on the way. You’ll have to seek the user’s permission using the method above to use any of the data types. To see a complete list of data types along with the required permissions, see this list here.
Now, to write data into Health Connect, you first need to create a record. This record contains data about particular health metrics, such as steps. Depending on the type, the record can be:
- Basic, such as steps.
- Unit of Measurement, such as Nutrition, measured in units of Mass like grams or kilocalories.
- Series Data, such as heart rate.
As an example, this is how you would write a steps record:
suspend fun insertSteps(healthConnectClient: HealthConnectClient) {
try {
val stepsRecord = StepsRecord(
count = 120,
startTime = Instant.now().minusSeconds(3600),
endTime = endTime = Instant.now(),
startZoneOffset = ZoneOffset.of("+05:30"),
endZoneOffset = ZoneOffset.of("+05:30"),
// Optional
metadata = Metadata(
dataOrigin = DataOrigin(
"Health Connect Wearable Device"
)
)
)
healthConnectClient.insertRecords(listOf(stepsRecord))
} catch (e: Exception) {
// Run error handling here
}
}
[add copy]
You can add an optional metadata field, containing information such as the source of data, an ID, or device information.
To read data, you can simply invoke the healthConnectClient giving it a range of time to fetch records for:
suspend fun readSteps(
healthConnectClient: HealthConnectClient,
) {
try {
val response = healthConnectClient.readRecords(
ReadRecordsRequest(
StepsRecord::class,
timeRangeFilter = TimeRangeFilter.between(Instant.now().minusSeconds(3600), Instant.now())
)
)
response.records.forEach {
// Do something
}
} catch (e: Exception) {
// Run error handling here
}
}
[add copy]
However, this data is raw, and the official platform guidance recommends reading aggregated data so that you don’t have to manually process and filter the records. Here’s how you can find the total distance that a user has walked in the past week, in meters:
suspend fun aggregateDistance(
healthConnectClient: HealthConnectClient,
) {
try {
val response = healthConnectClient.aggregate(
AggregateRequest(
metrics = setOf(DistanceRecord.DISTANCE_TOTAL),
timeRangeFilter = TimeRangeFilter.between(Instant.now().minusSeconds(604800), Instant.now())
)
)
val distanceTotalInMeters = response[DistanceRecord.DISTANCE_TOTAL]?.inMeters ?: 0L
} catch (e: Exception) {
// Run error handling here
}
}
[add copy]
The best part perhaps is that you can simply synchronize data between Health Connect and your app, hence obtaining differential data, making it easier for you to aggregate data from multiple streams. However, syncing is somewhat complex and may be difficult to scale for multiple data types. For more details, check out this guide.
Wrapping Up
Wrapping up, Health Connect is Google's answer to a unified health data management system on Android. It's designed to make life easier for developers by providing a single interface for health and fitness data, drawing inspiration from the likes of Apple's HealthKit, albeit with its own unique features and improvements.
This change means developers will need to familiarize themselves with this new platform, rethinking their strategies for handling health data, and potentially revising their existing app architectures.
For users, Health Connect offers the prospect of having their health data from different apps and devices all neatly gathered in one spot. It pledges enhanced control over this data with improved privacy. Now, while this all sounds very promising, it's early days yet. The platform is still fresh on the scene and has some big shoes to fill with the upcoming deprecation of FitBit API. So, keep an eye out for how things pan out and be ready to adapt and evolve along with this new health data landscape on Android. It's an exciting time indeed!